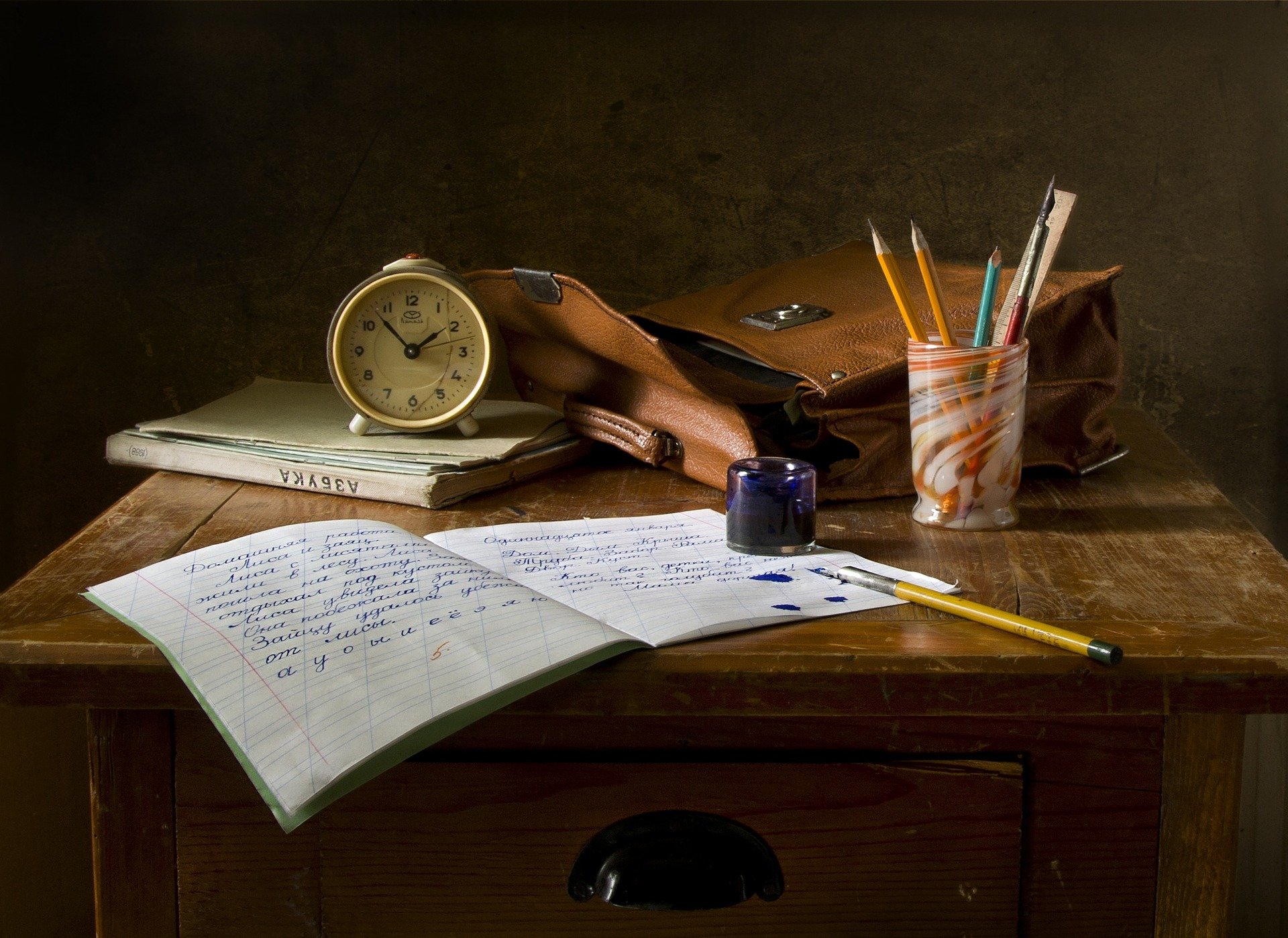
Tag: Algorithms
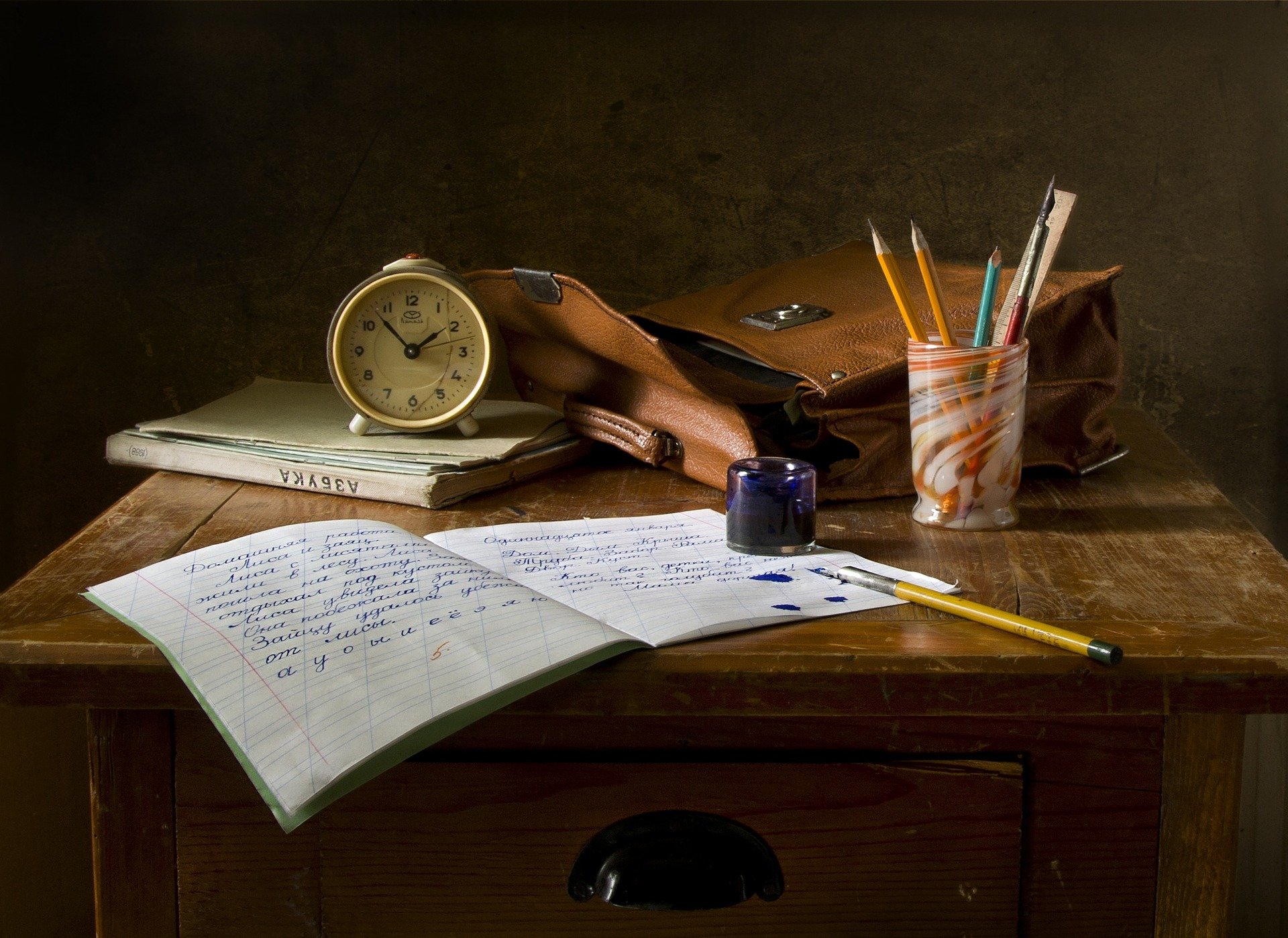
Problem A “tuple” is defined as an ordered collection of elements which can include duplicate values and each element is assigned an order. A tuple with n elements (a1, a2, a3, ..., an) can be expressed using the set notation in various ways, with any ordering of the subsets as:
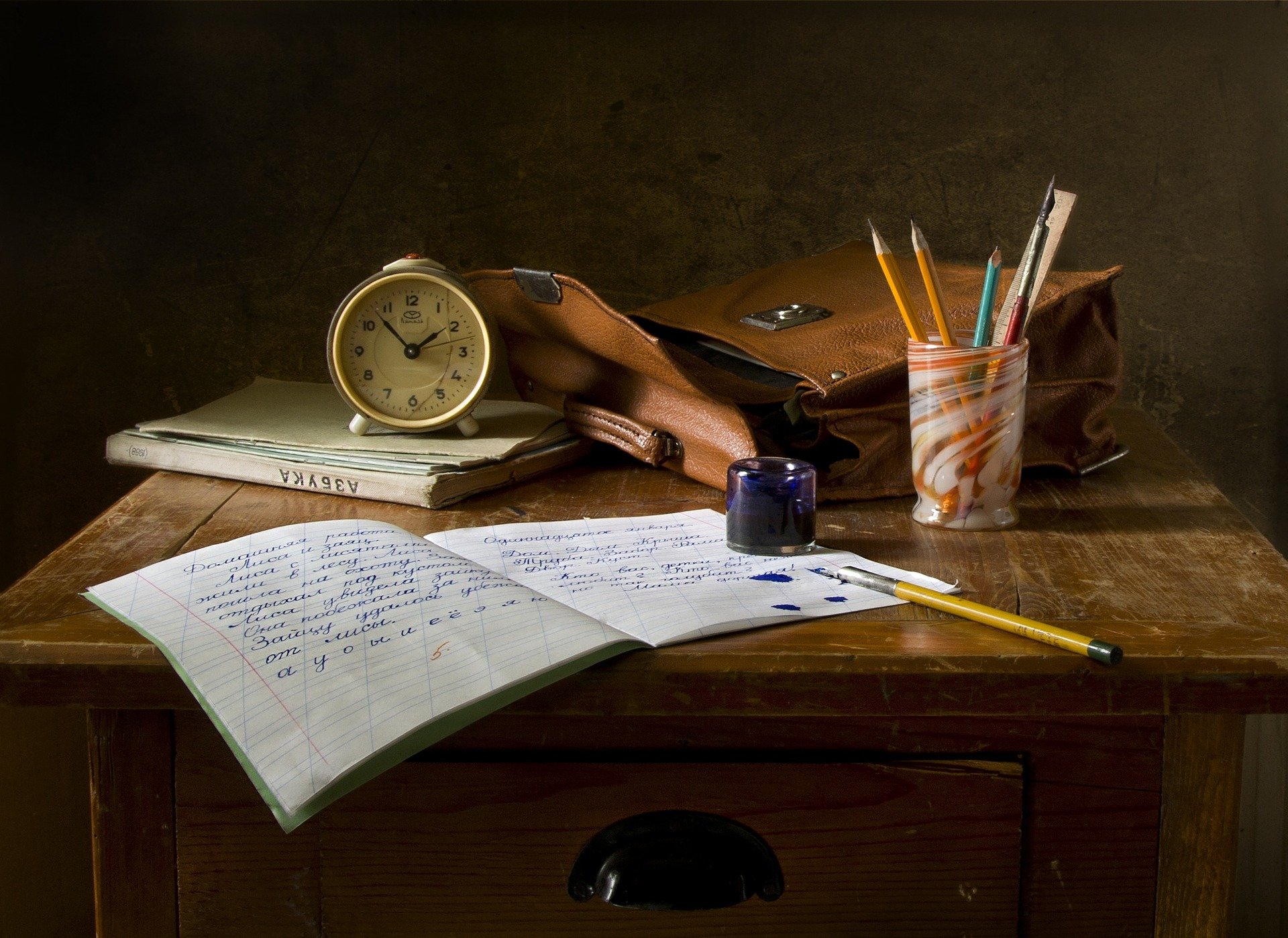
Problem Define a string as a “valid bracket string” if it follows the given rules:
“()”, “[]”, and “{}” are all valid bracket strings. If A is a valid bracket string, then “(A)”, “[A]”, and “{A}” are also valid bracket strings. For example, since “[]” is a valid bracket string, “([])” is also valid.
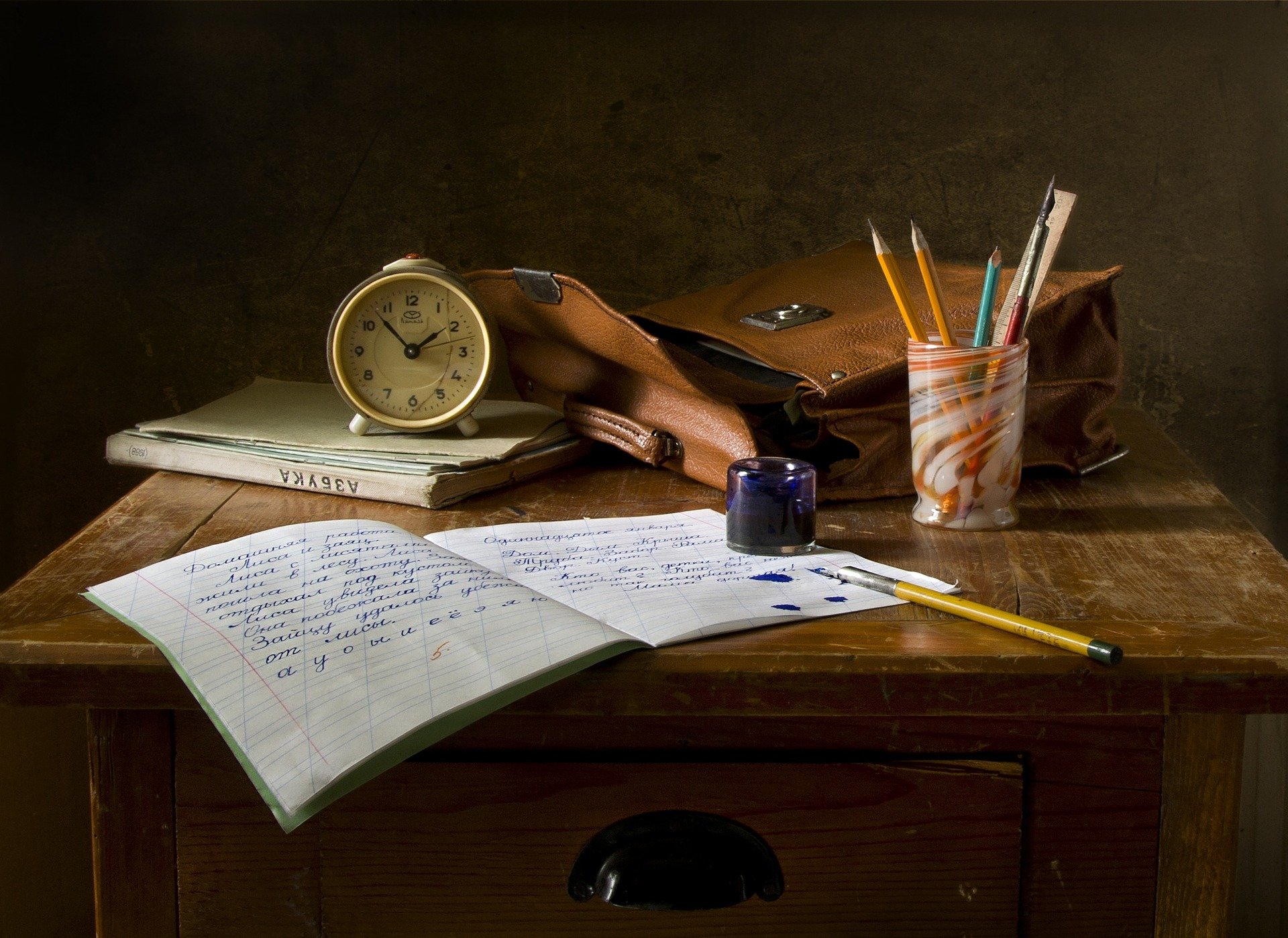
Problem In the Jay’s game tournament, N players compete in a knockout format. Each player is sequentially assigned a number from 1 to N. The matches are arranged as 1 vs 2, 3 vs 4, …, N-1 vs N. The winner of each game advances to the next round, where they receive new sequential numbers starting from 1 for the advancing players.
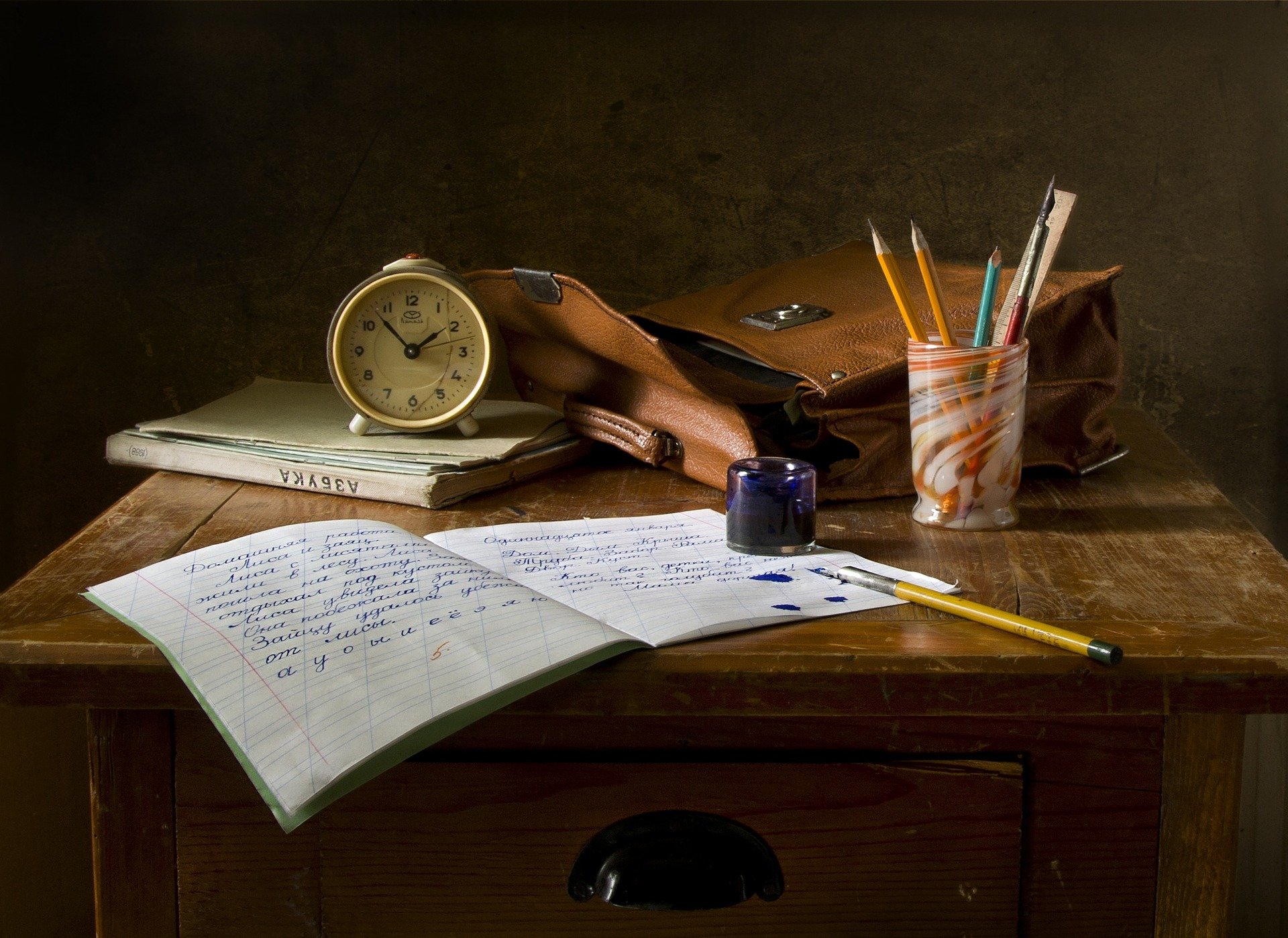
Problem Several people are playing the game of English word chain where each player takes turns saying a word that must begin with the last letter of the previous word. Here are the rules:
Players take turns in a sequence from 1 to n. After the last player, it starts again with the first player.
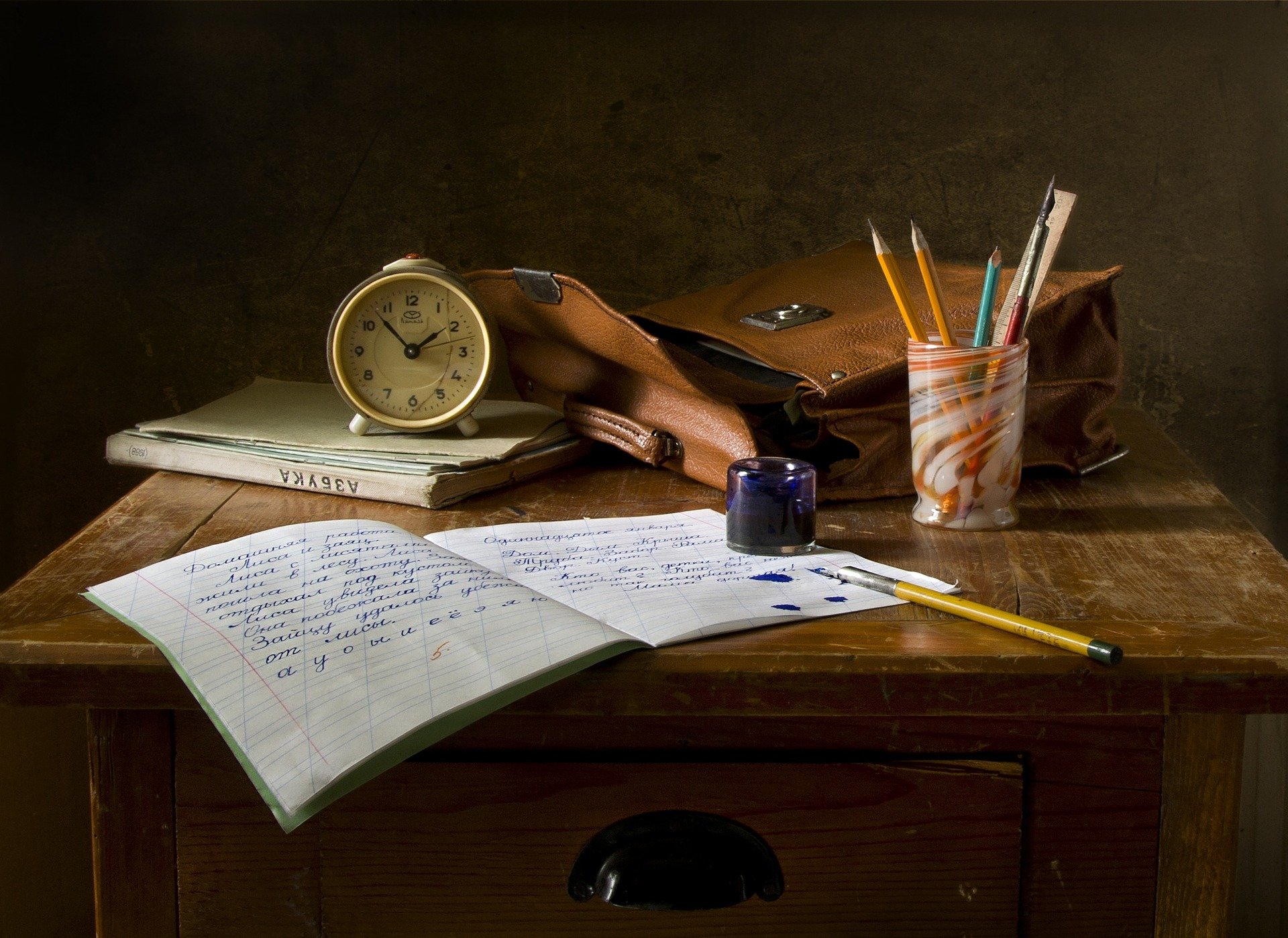
Problem Given a number, your task is to remove k digits from the number in such a way that the remaining digits form the largest possible number. The number is provided as a string number and the integer k represents the number of digits to remove. Your function solution should return the resulting largest number as a string.
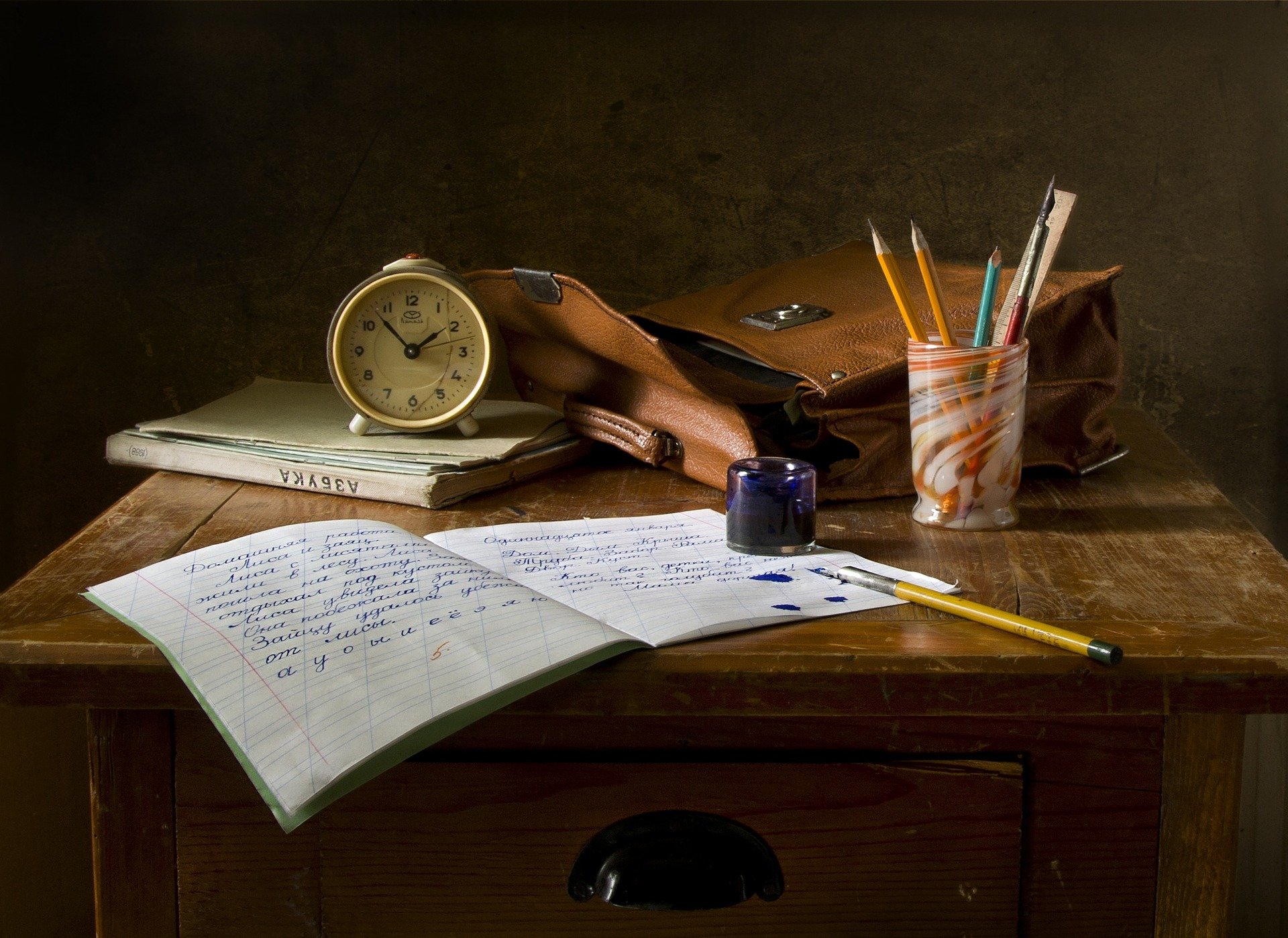
Problem In the ROR game, you play on a team that competes to destroy the opposing team’s base first, making it advantageous to reach the enemy base as quickly as possible. Suppose you’re a team member and are located at position (row: 1, column: 1) on a 5x5 map, while the enemy base is at (row: 5, column: 5).
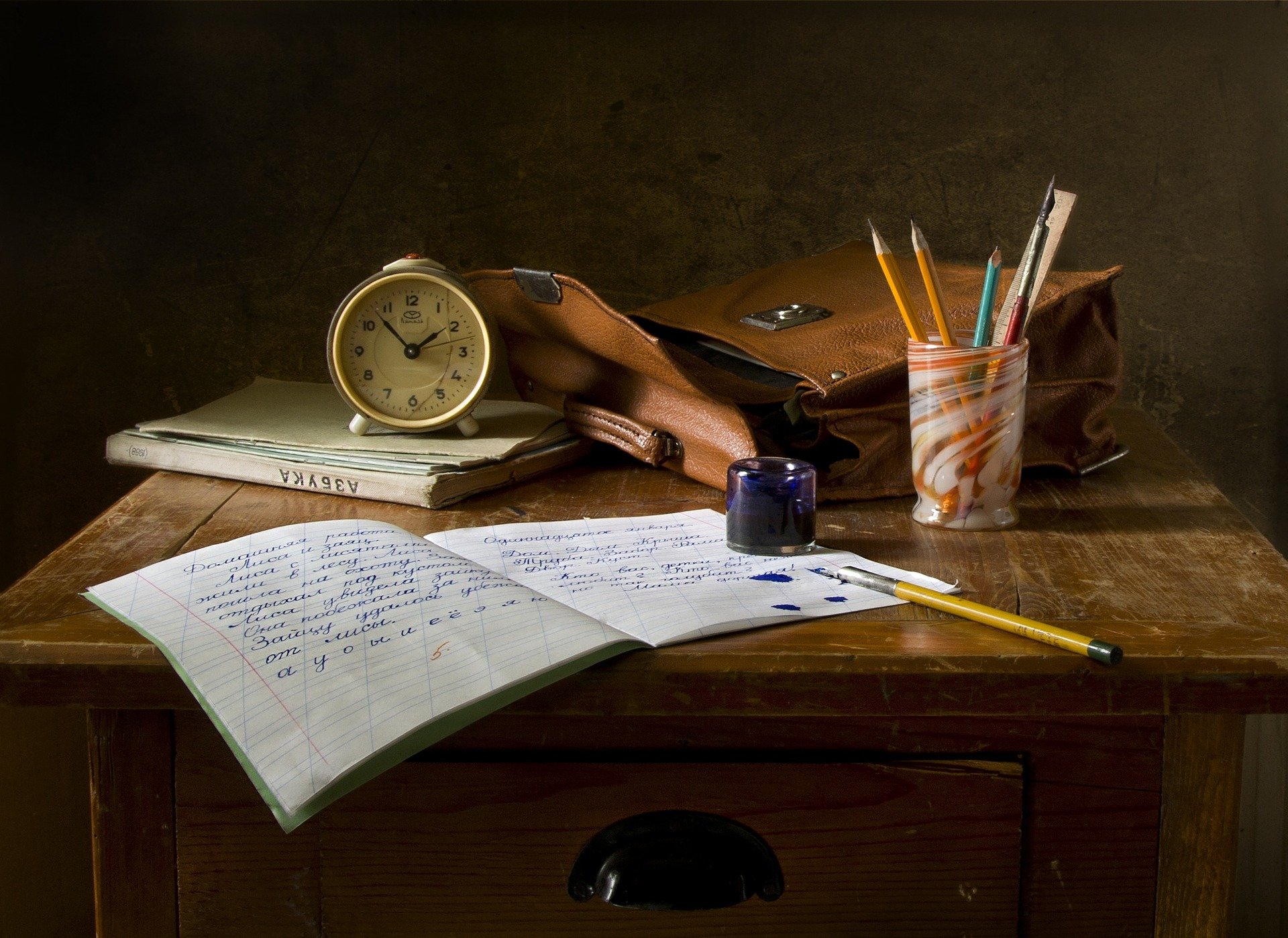
Problem You are given an array of non-negative integers and a target number. Your task is to find how many ways you can add and subtract the given numbers to achieve the target number. The order of numbers in the array must be preserved. For example, with the array [1, 1, 1, 1, 1], there are five ways to reach the target number 3:
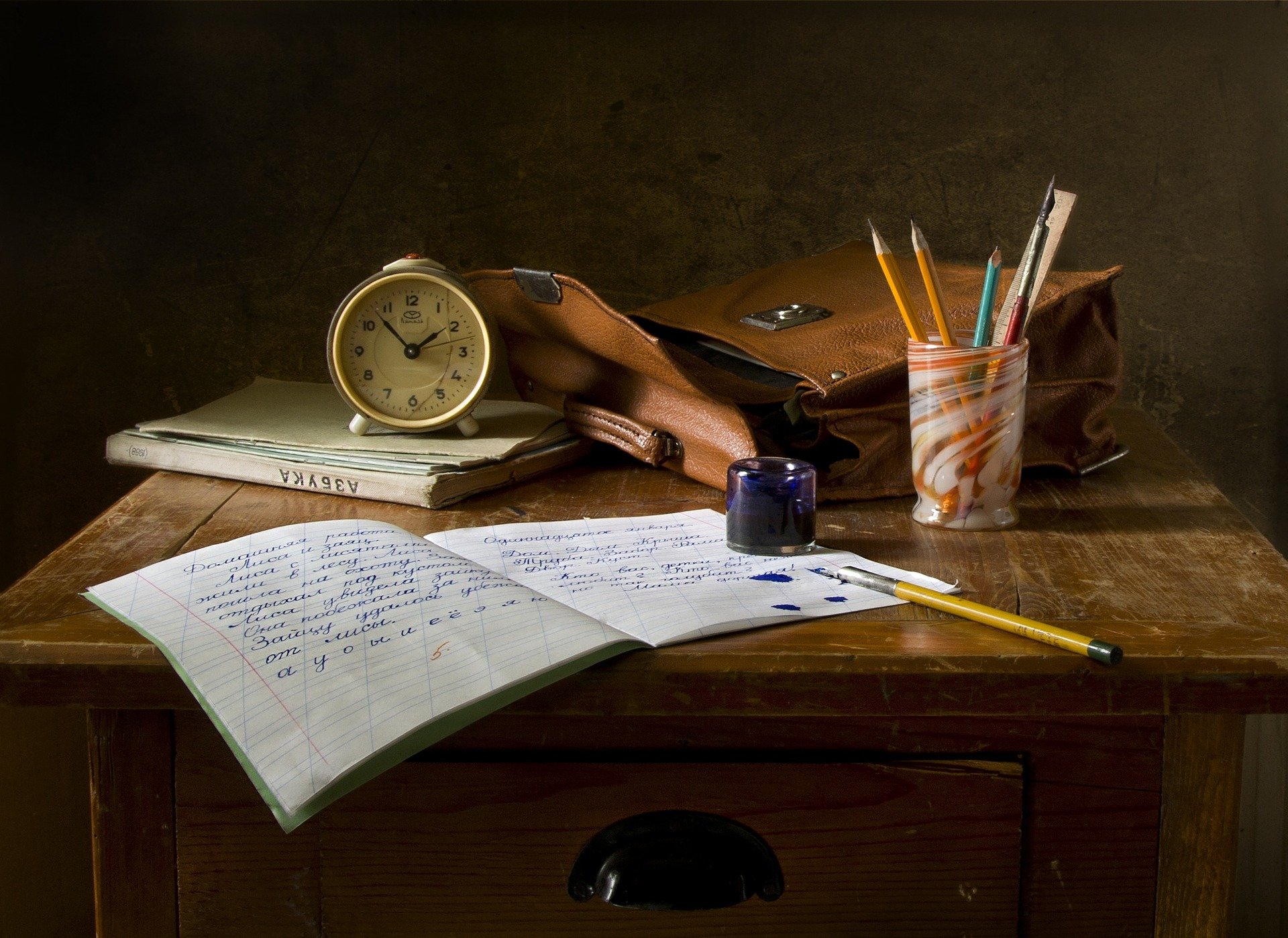
Problem In a power grid network composed of n transmission towers connected in a tree structure via wires, you are tasked with dividing the network into two parts by cutting one of the wires. The goal is to make the number of transmission towers in each part as equal as possible.
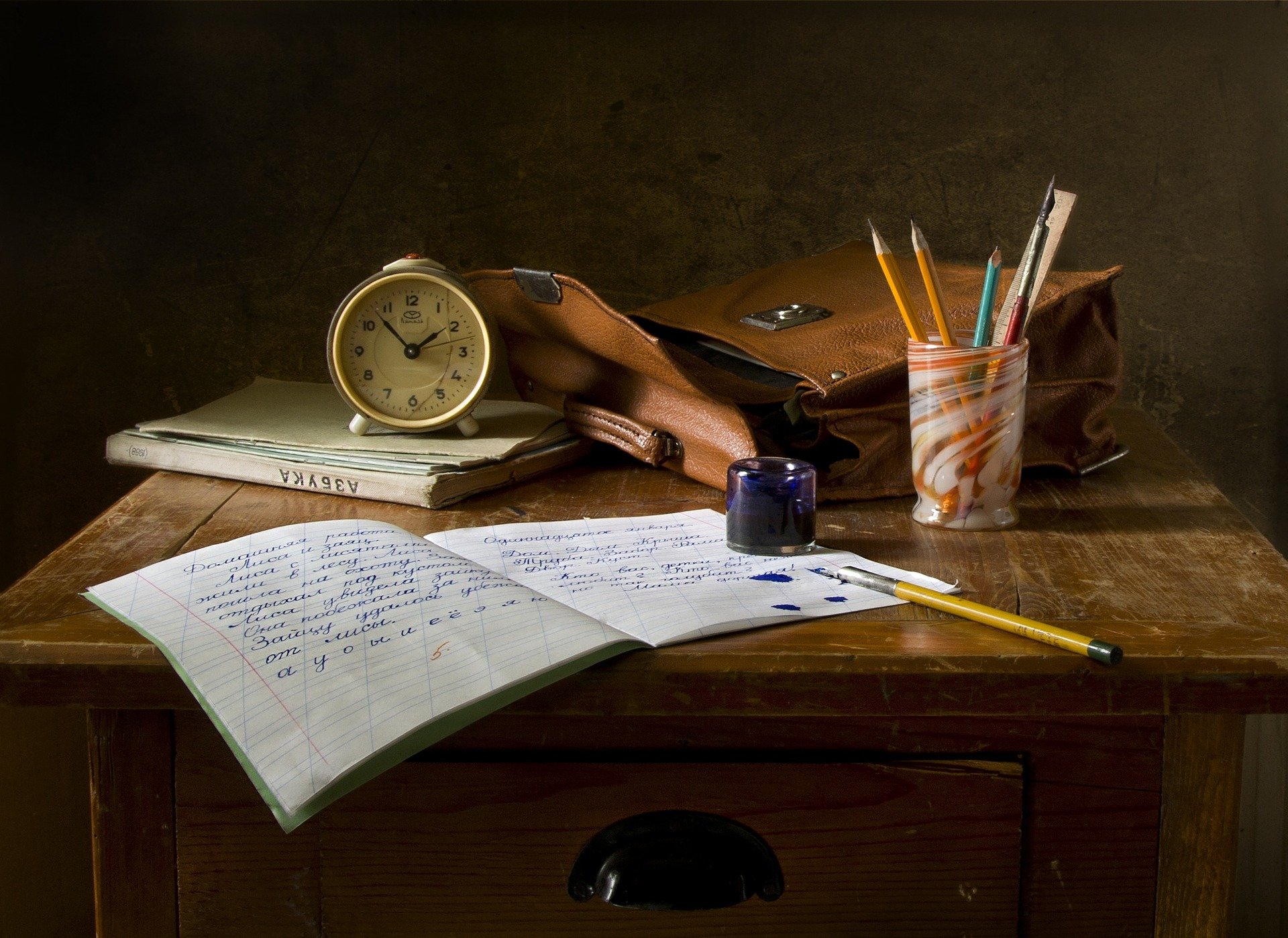
Problem In the XX game, there is a fatigue system represented by non-negative integers, allowing players to explore dungeons by using a certain amount of fatigue. Each dungeon requires a “minimum required fatigue” to start exploring and consumes a certain amount of “fatigue” upon completion. For example, to explore a dungeon with a “minimum required fatigue” of 80 and a “consumption fatigue” of 20, a player’s current remaining fatigue must be at least 80, and it will decrease by 20 after exploring the dungeon.
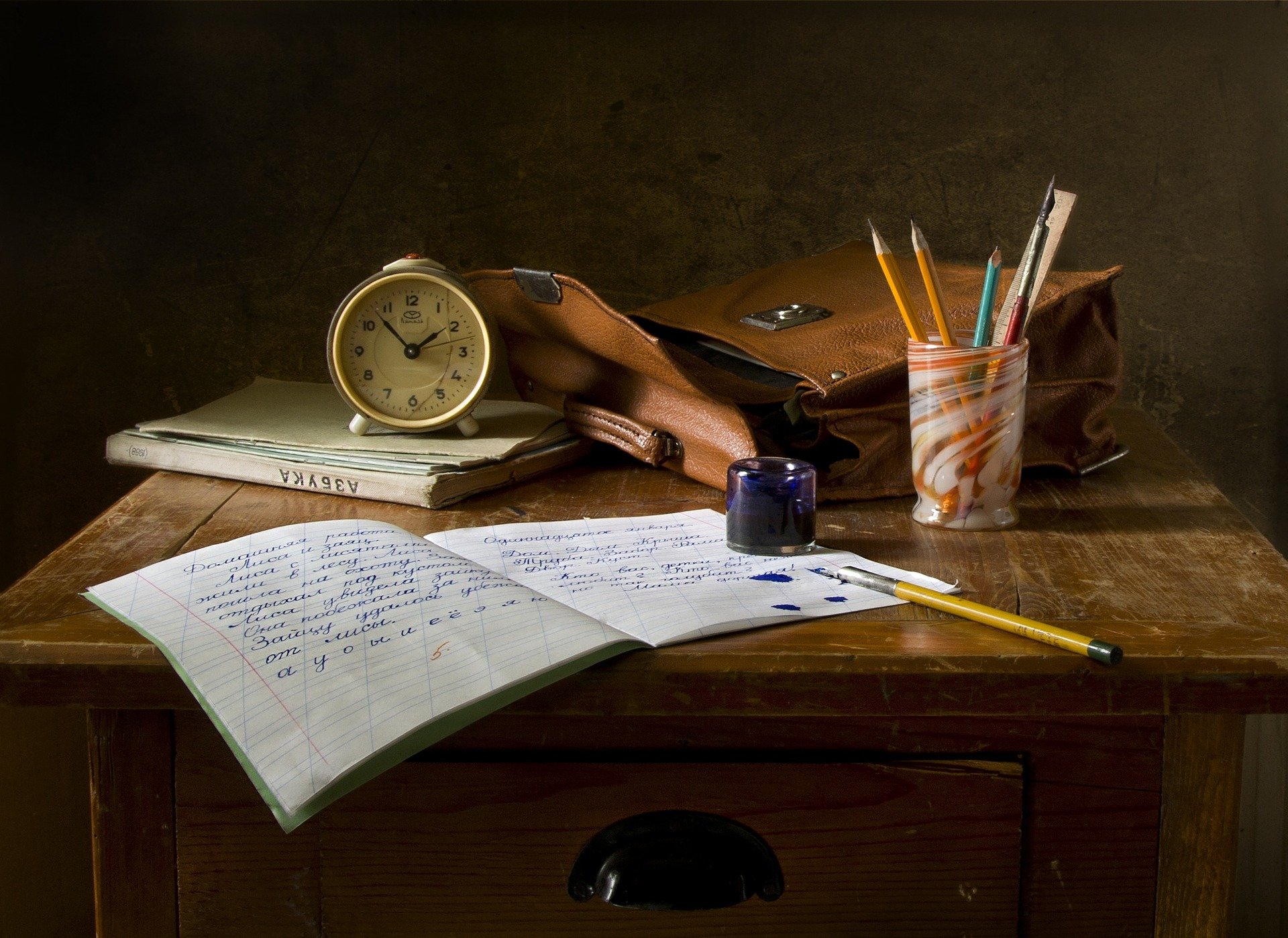
Problem The Programmers team is currently performing feature improvement tasks. Each feature can only be released when its progress reaches 100%. However, since the development speed of each feature varies, it is possible for features developed later to be completed earlier. In such cases, a feature that is developed later will be released together with the feature in front of it that is scheduled for earlier release.
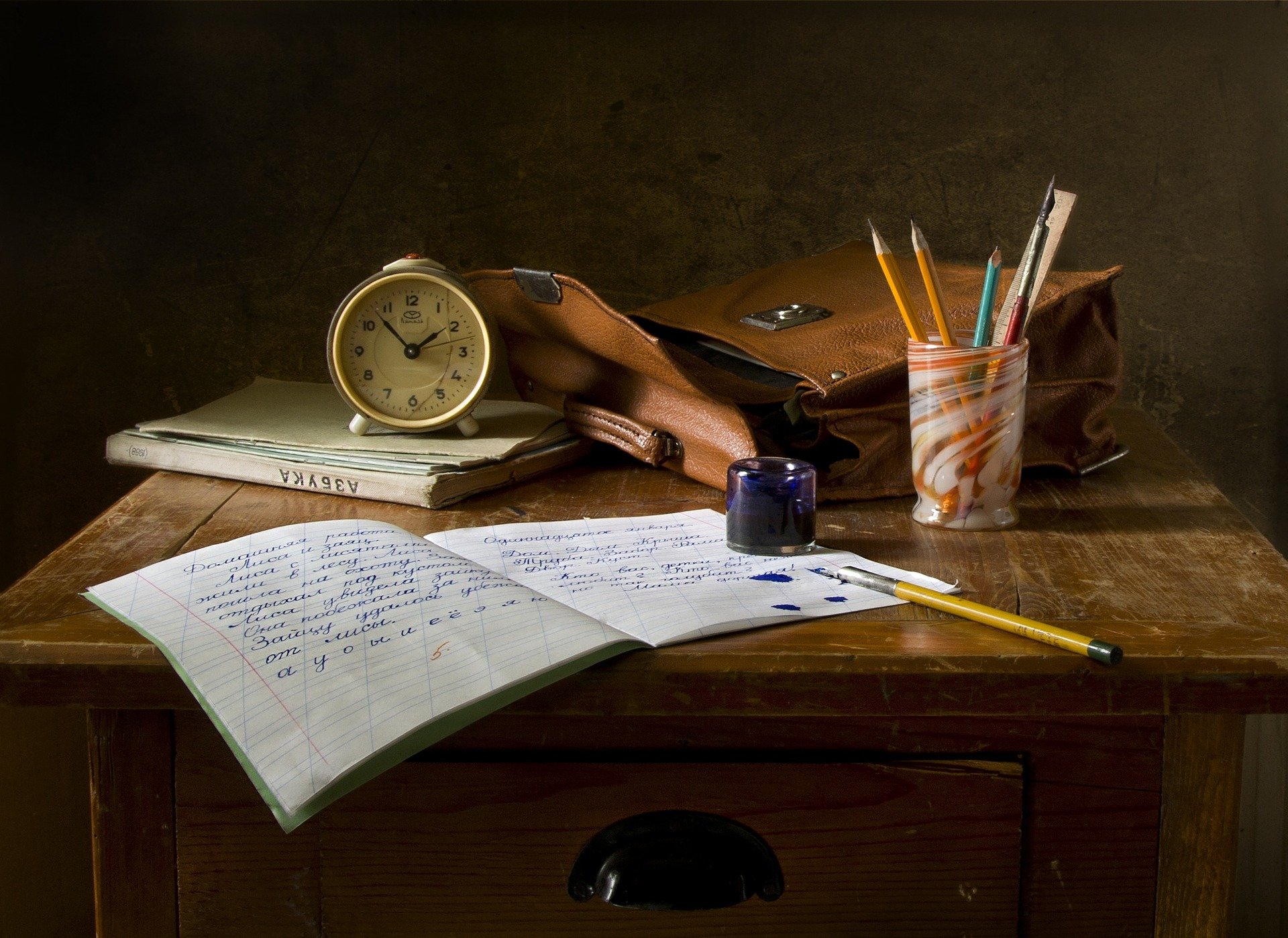
Problem Given an array arr consisting of integers ranging from 0 to 9, the task is to remove consecutive duplicates while preserving the order of the remaining elements. For instance, if arr = [1, 1, 3, 3, 0, 1, 1], the result should be [1, 3, 0, 1]. Similarly, for arr = [4, 4, 4, 3, 3], the result should be [4, 3].
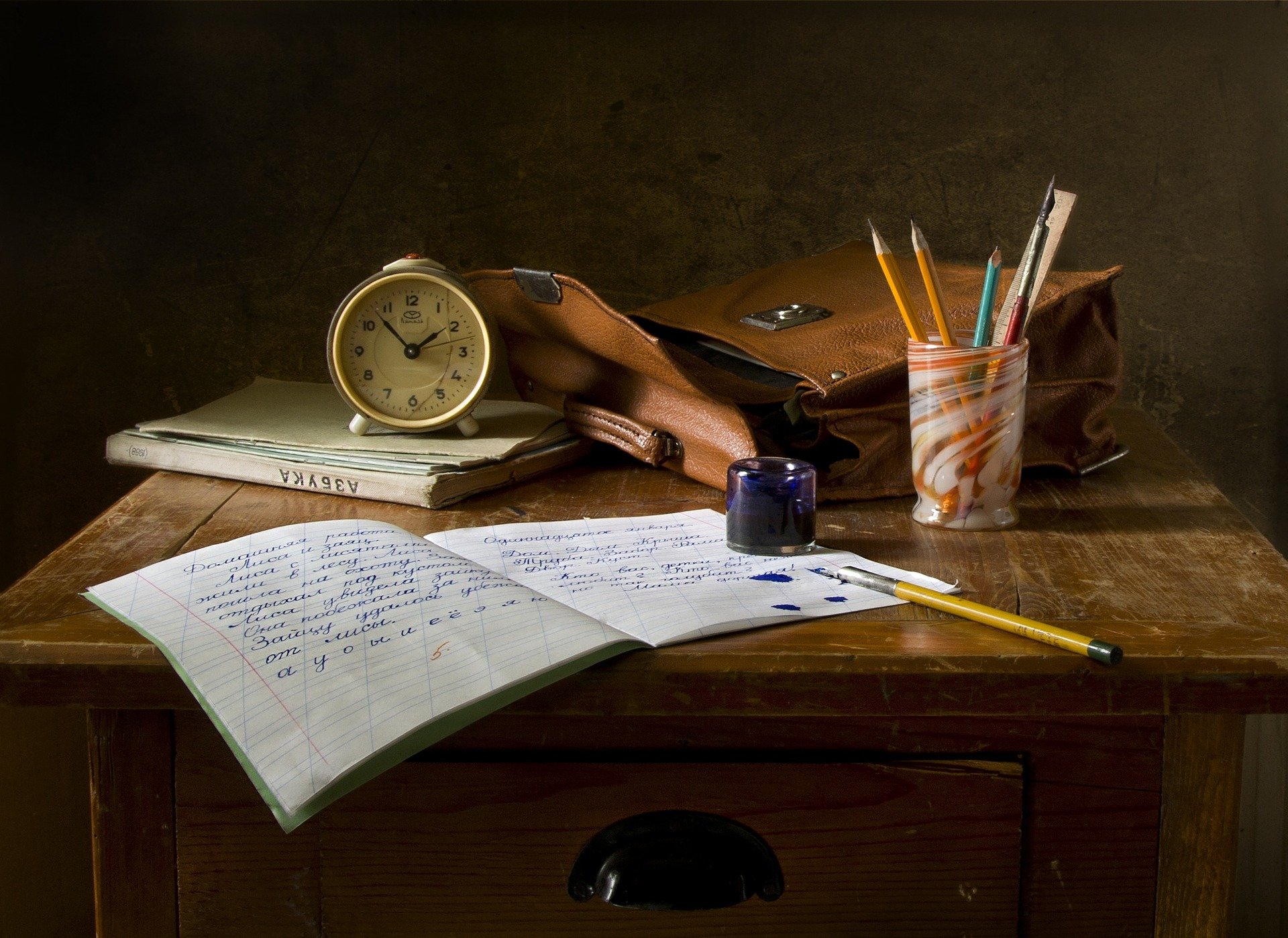
Problem The H-Index is a metric that measures the productivity and impact of a scientist’s research. It represents the highest number h such that the scientist has published h papers that have each been cited at least h times. According to Wikipedia, the H-Index is calculated as follows:
For a given scientist who has published n papers, if h of these papers have at least h citations each, and the other papers have no more than h citations each, then h is the maximum value for this scientist’s H-Index.
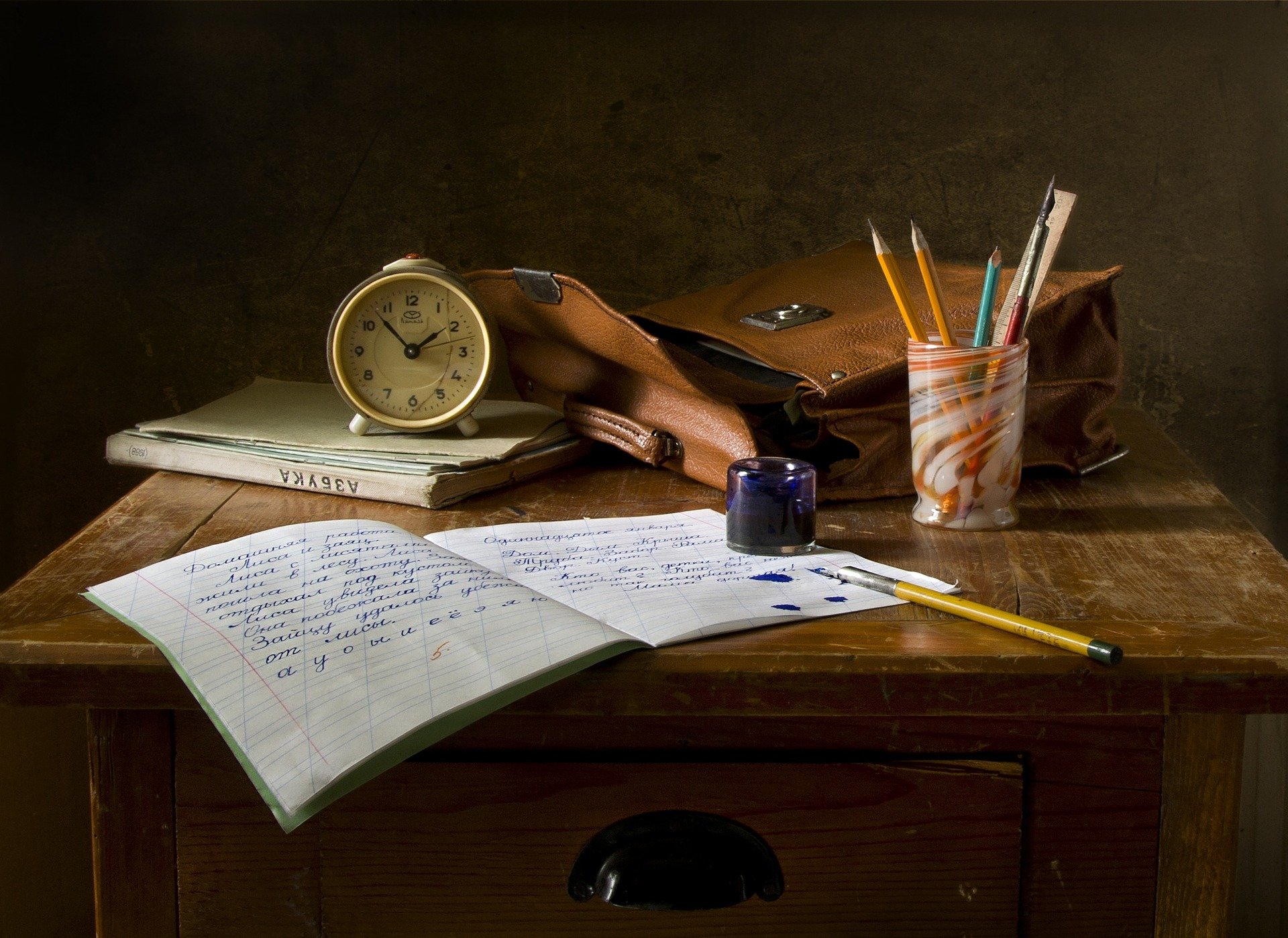
Problem Given an array of non-negative integers, your task is to determine the largest number that can be formed by concatenating the integers together. For example, with the integers [6, 10, 2], you can form the numbers [6102, 6210, 1062, 1026, 2610, 2106] through different arrangements. The largest number from these combinations is 6210.
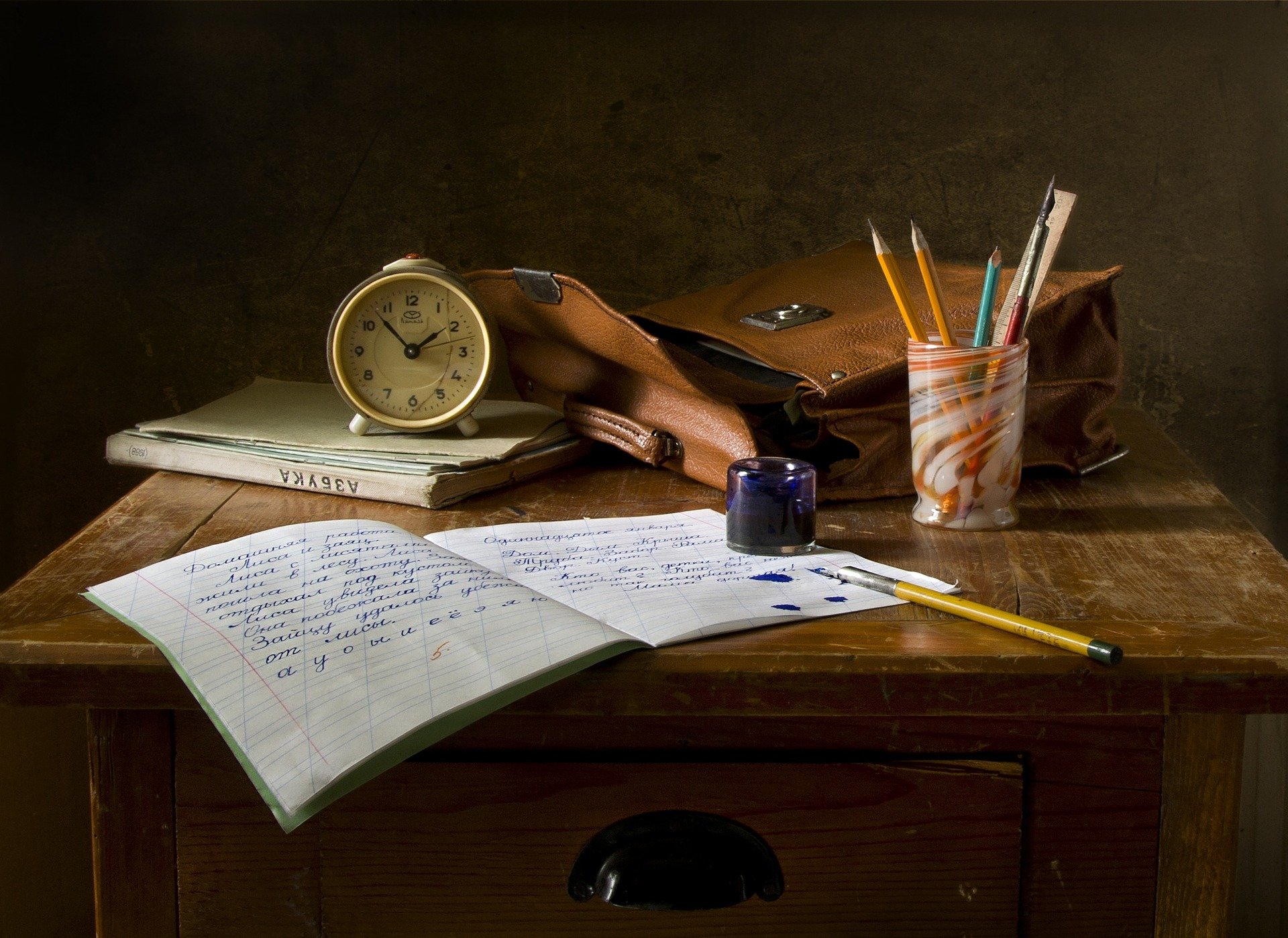
Problem To find a number at the k-th position after slicing and sorting the array from the i-th to j-th number, consider the following: For example, if the array is [1, 5, 2, 6, 3, 7, 4], i = 2, j = 5, and k = 3, slicing the array from the 2nd to the 5th number yields [5, 2, 6, 3].
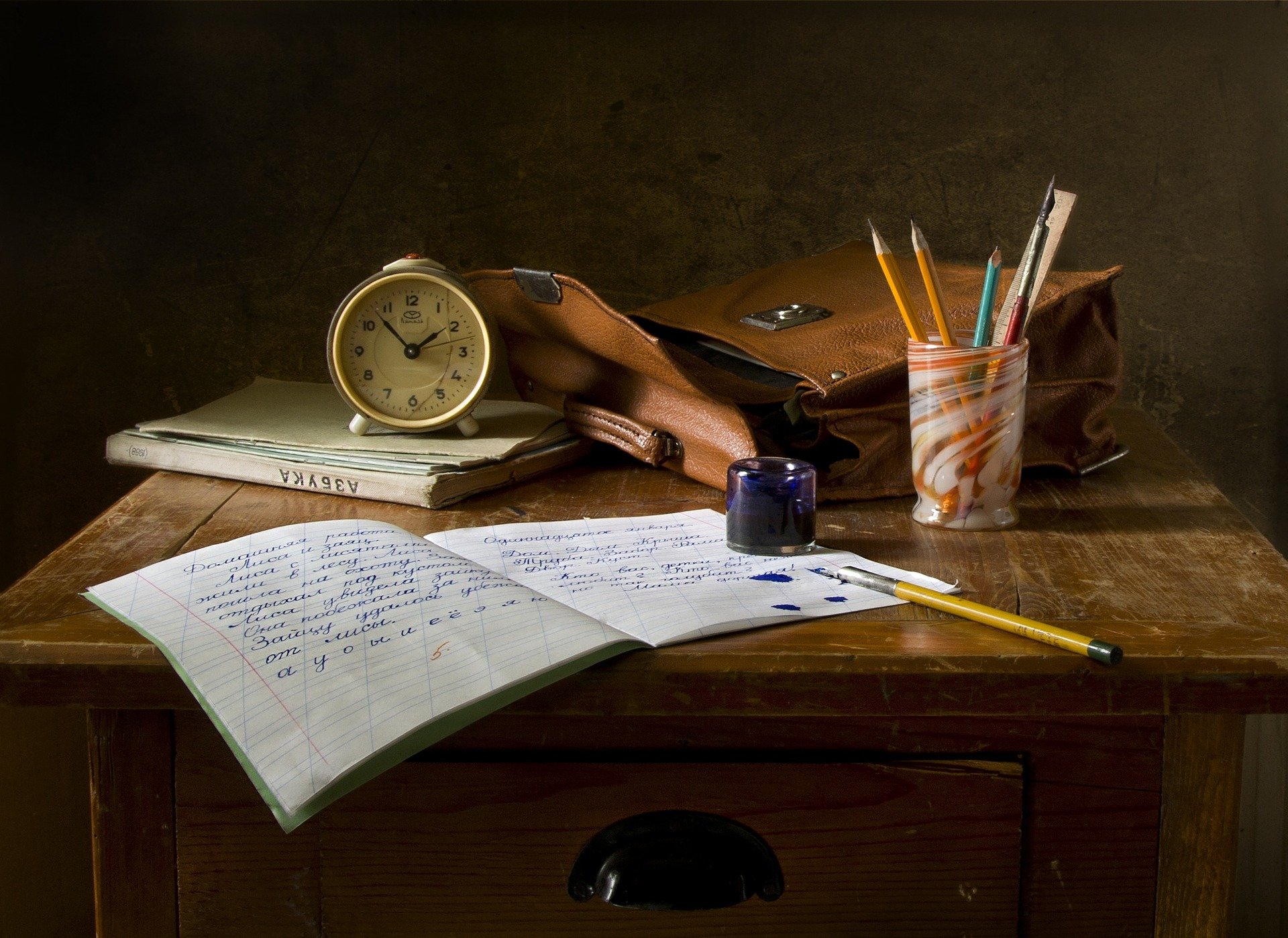
Problem Jay likes sequences, so he use to playing with a sequence of size N. He will transform the sequence according to K queries, and the format and process of the queries are as follows:
L R X: Let’s say the sequence sorted in ascending order is A[1], A[2], …, A[N].
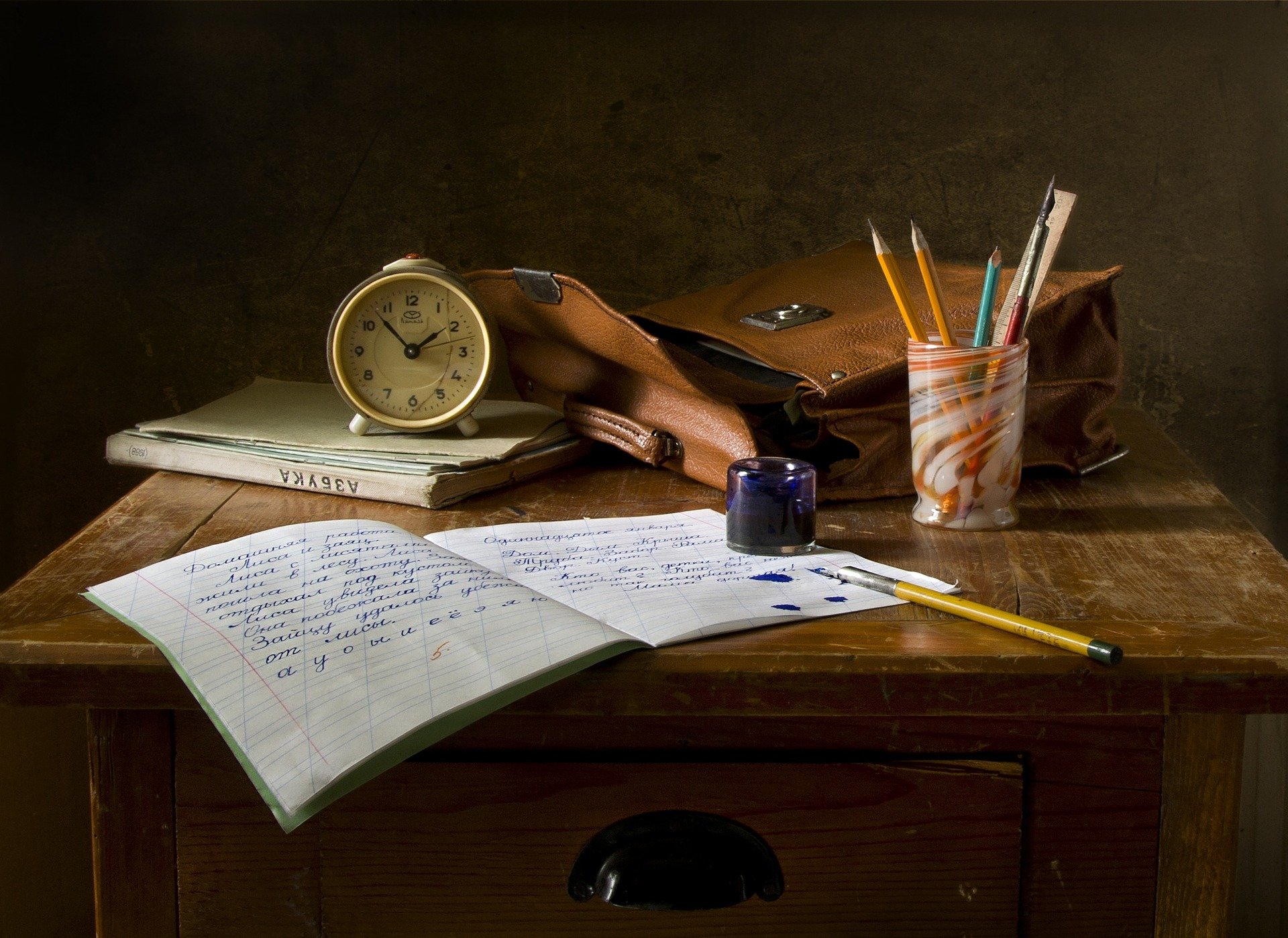
Introduction What this post focus on This posting will cover almost every concept of How to sort (primary). Plus, I’ll post realistic algorithm problem of our real world so that you can apply those concept to real world problem and learn how to use it. I really hope so. Let’s see the below example.
Table of Contents
latest Posts
tuple
Problem A “tuple” is defined as an ordered collection of elements which can include duplicate values and each element is assigned an order. A tuple with n elements (a1, a2, a3, ..., an) can be expressed using the set notation in various ways, with any ordering of the subsets as:
2024-5-1
braket rotating
Problem Define a string as a “valid bracket string” if it follows the given rules:
“()”, “[]”, and “{}” are all valid bracket strings. If A is a valid bracket string, then “(A)”, “[A]”, and “{A}” are also valid bracket strings. For example, since “[]” is a valid bracket string, “([])” is also valid.
2024-4-27
Tournament
Problem In the Jay’s game tournament, N players compete in a knockout format. Each player is sequentially assigned a number from 1 to N. The matches are arranged as 1 vs 2, 3 vs 4, …, N-1 vs N. The winner of each game advances to the next round, where they receive new sequential numbers starting from 1 for the advancing players.
2024-4-25
Last and First
Problem Several people are playing the game of English word chain where each player takes turns saying a word that must begin with the last letter of the previous word. Here are the rules:
Players take turns in a sequence from 1 to n. After the last player, it starts again with the first player.
2024-4-23
High Score Kit: Greedy#1
Problem Given a number, your task is to remove k digits from the number in such a way that the remaining digits form the largest possible number. The number is provided as a string number and the integer k represents the number of digits to remove. Your function solution should return the resulting largest number as a string.
2024-4-11
High Score Kit: DFS&BFS#2
Problem In the ROR game, you play on a team that competes to destroy the opposing team’s base first, making it advantageous to reach the enemy base as quickly as possible. Suppose you’re a team member and are located at position (row: 1, column: 1) on a 5x5 map, while the enemy base is at (row: 5, column: 5).
2024-4-11